abstract class는 abstract
로 선언된 클래스 입니다 - abstract 메소드들이 포함될 수도 포함하지 않을 수도 있습니다.
abstract class들은 인스턴스화 될 수 없지만 subclass는 될 수 있습니다..
abstract method는 아래와 같이 구현 없이(중괄호 없이 semicolon만 있는) 선언된 메소드 입니다:
An abstract class is a class that is declared abstract
—it may or may not include abstract methods. Abstract classes cannot be instantiated, but they can be subclassed.
An abstract method is a method that is declared without an implementation (without braces, and followed by a semicolon), like this:
abstract void moveTo(double deltaX, double deltaY);
클래스에 추상 메소드가 포함되면, 클래스는 abstract로 선언되어야 합니다:
If a class includes abstract methods, then the class itself must be declared abstract
, as in:
public abstract class GraphicObject {
// declare fields
// declare nonabstract methods
abstract void draw();
}
abstract 클래스가 subclass되는 경우, subclass는 통상적으로 그 부모 클래스 안에 모든 추상 메소드들에 대한 구현을 제공합니다.
구현을 제공하지 않는 경우에는 그 subclass가 abstract
로 선언되어야 합니다.
When an abstract class is subclassed, the subclass usually provides implementations for all of the abstract methods in its parent class. However, if it does not, then the subclass must also be declared abstract
.
참고: 디폴트 또는 static으로 선언되지 않은, interface (Interfaces 섹션 참조) 안의 메소드들은 묵시적으로 abstract 인데, 따라서
abstract
modifier는 interface methods와는 사용되지 않습니다(사용될 수는 있으나 필요하지 않습니다).Note: Methods in an interface (see the Interfaces section) that are not declared as default or static are implicitly abstract, so the
abstract
modifier is not used with interface methods. (It can be used, but it is unnecessary.)추상 클래스는 인터페이스와 비슷합니다.
추상 클래스는 인스턴스를 만들 수 없으며, 추상 클래스에는 구현되거나 구현되지 않은 선언된 메소드의 혼합이 포함될 수 있습니다.
하지만, 추상 클래스에서는, static과 final이 아닌 필드를 선언할 수 있으며, public, protected, private의 구체적 메소드들을 정의할 수 있습니다.
인터페이스에서는, 모든 필드는 자동으로 public, static, final이며, (기본 메소드로) 선언하거나 정의하는 모든 메소드들은 public입니다.
추상이든 아니된 추가로 1개의 클래스 만을 확장할 수 있는데, 인터페이스는 제한 없이 구현할 수 있습니다.
추상 클래스 또는 인터페이스를 어느 것을 사용해야 합니까?
Abstract classes are similar to interfaces. You cannot instantiate them, and they may contain a mix of methods declared with or without an implementation. However, with abstract classes, you can declare fields that are not static and final, and define public, protected, and private concrete methods. With interfaces, all fields are automatically public, static, and final, and all methods that you declare or define (as default methods) are public. In addition, you can extend only one class, whether or not it is abstract, whereas you can implement any number of interfaces.
Which should you use, abstract classes or interfaces?
- Consider using abstract classes if any of these statements apply to your situation:
- You want to share code among several closely related classes.
- You expect that classes that extend your abstract class have many common methods or fields, or require access modifiers other than public (such as protected and private).
- You want to declare non-static or non-final fields. This enables you to define methods that can access and modify the state of the object to which they belong.
- Consider using interfaces if any of these statements apply to your situation:
- You expect that unrelated classes would implement your interface. For example, the interfaces
Comparable
andCloneable
are implemented by many unrelated classes. - You want to specify the behavior of a particular data type, but not concerned about who implements its behavior.
- You want to take advantage of multiple inheritance of type.
- You expect that unrelated classes would implement your interface. For example, the interfaces
An example of an abstract class in the JDK is AbstractMap
, which is part of the Collections Framework. Its subclasses (which include HashMap
, TreeMap
, and ConcurrentHashMap
) share many methods (including get
, put
, isEmpty
,containsKey
, and containsValue
) that AbstractMap
defines.
An example of a class in the JDK that implements several interfaces is HashMap
, which implements the interfaces Serializable
, Cloneable
, and Map<K, V>
. By reading this list of interfaces, you can infer that an instance of HashMap
(regardless of the developer or company who implemented the class) can be cloned, is serializable (which means that it can be converted into a byte stream; see the section Serializable Objects), and has the functionality of a map. In addition, the Map<K, V>
interface has been enhanced with many default methods such as merge
and forEach
that older classes that have implemented this interface do not have to define.
Note that many software libraries use both abstract classes and interfaces; the HashMap
class implements several interfaces and also extends the abstract class AbstractMap
.
추상 클래스 예제 An Abstract Class Example
객체-지향 drawing application에서, circles, rectangles, lines, Bezier curves, 그리고 많은 다른 graphic objects를 그릴 수 있습니다.
이들 객체들은 모두 어떤 상태(예: position, orientation, line color, fill color)와 동작(예: moveTo, rotate, resize, draw)을 공통으로 갖고 있습니다.
이들 상태와 동작의 일부는 모든 그래픽 객체에 대하여 동일합니다(예: position, fill color, and moveTo).
다른 것들은 서로 다른 구현을 요구합니다(예, resize or draw).
모든 GraphicObject
들은 자신을 draw 또는 resize 할 수 있어야 합니다; 그들은 단지 그것을 하는 방법에서만 차이가 있습니다.
이것은 abstract superclass의 완벽한 상황입니다.
아래 그림에 있는 것처럼(예, GraphicObject
) 유사성을 활용할 수 있으며 동일한 추상 부모 객체로부터 상속받기 위하여 모든 그래픽 객체들을 선언할 수 있습니다.
In an object-oriented drawing application, you can draw circles, rectangles, lines, Bezier curves, and many other graphic objects. These objects all have certain states (for example: position, orientation, line color, fill color) and behaviors (for example: moveTo, rotate, resize, draw) in common. Some of these states and behaviors are the same for all graphic objects (for example: position, fill color, and moveTo). Others require different implementations (for example, resize or draw). AllGraphicObject
s must be able to draw or resize themselves; they just differ in how they do it. This is a perfect situation for an abstract superclass. You can take advantage of the similarities and declare all the graphic objects to inherit from the same abstract parent object (for example, GraphicObject
) as shown in the following figure.
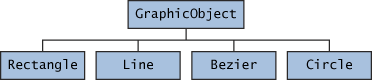
Classes Rectangle, Line, Bezier, and Circle Inherit from GraphicObject
First, you declare an abstract class, GraphicObject
, to provide member variables and methods that are wholly shared by all subclasses, such as the current position and the moveTo
method. GraphicObject
also declares abstract methods for methods, such as draw
or resize
, that need to be implemented by all subclasses but must be implemented in different ways. The GraphicObject
class can look something like this:
abstract class GraphicObject {
int x, y;
...
void moveTo(int newX, int newY) {
...
}
abstract void draw();
abstract void resize();
}
Each nonabstract subclass of GraphicObject
, such as Circle
and Rectangle
, must provide implementations for the draw
and resize
methods:
class Circle extends GraphicObject {
void draw() {
...
}
void resize() {
...
}
}
class Rectangle extends GraphicObject {
void draw() {
...
}
void resize() {
...
}
}
When an Abstract Class Implements an Interface
Interfaces
섹션에서 interface를 구현하는 클래스는 interface's methods를 모두 구현해야 한다고 강조되었습니다.
하지만 클래스가 abstract로 선언된다는 전제하에 interface's methods를 모두 구현하지 않는 클래스를 정의할 수도 있습니다.
In the section on Interfaces
, it was noted that a class that implements an interface must implement all of the interface's methods. It is possible, however, to define a class that does not implement all of the interface's methods, provided that the class is declared to be abstract
. For example,
abstract class X implements Y {
// implements all but one method of Y
}
class XX extends X {
// implements the remaining method in Y
}
위의 경우, 클래스 X는 추상적이어야 하는데 그 이유는 클래스 X가 Y를 모두 구현하지 않기 때문이며, 하지만 실제로는 클래스 XX가 Y를 구현합니다.
In this case, class X
must be abstract
because it does not fully implement Y
, but class XX
does, in fact, implement Y
.
Class Members
An abstract class may have static
fields and static
methods. You can use these static members with a class reference (for example, AbstractClass.staticMethod()
) as you would with any other class.
'Java 배우기' 카테고리의 다른 글
Questions and Exercises: Inheritance 질문과 연습: 상속 (0) | 2016.01.05 |
---|---|
Summary of Inheritance 상속 요약 (0) | 2016.01.05 |
Writing Final Classes and Methods Final 클래스 및 메소드 작성하기 (0) | 2016.01.05 |
Object as a Superclass 수퍼클래스로서의 객체 (0) | 2016.01.05 |
Using the Keyword super 키워드 super 사용하기 (0) | 2016.01.05 |
댓글