잘 알다시피, 클래스는 객체에 대한 설계도입니다; 어떤 클래스로부터 어떤 객체 1개를 생성합니다.
CreateObjectDemo
프로그램에서 취한 다음 명령문의 각각은, 객체를 생성하고 생성된 객체를 변수에 할당합니다:
As you know, a class provides the blueprint for objects; you create an object from a class. Each of the following statements taken from the CreateObjectDemo
program creates an object and assigns it to a variable:
Point originOne = new Point(23, 94); Rectangle rectOne = new Rectangle(originOne, 100, 200); Rectangle rectTwo = new Rectangle(50, 100);
첫째 라인은 클래스 Point
의 객체를 생성하며, 둘째 및 셋째 라인은 각각 클래스 Rectangle
의 객체를 생성합니다.
이들 명령문의 각각은 3개 부분을 갖고 있습니다 (상세한 것은 아래에서 논의):
The first line creates an object of the Point
class, and the second and third lines each create an object of the Rectangle
class.
Each of these statements has three parts (discussed in detail below):
- 선언: 굵은 글씨의 코드집합은 변수이름을 객체타입과 연계시킨 변수선언입니다.
Declaration: The code set in bold are all variable declarations that associate a variable name with an object type. - 인스턴스화: 키워드 new는 객체를 생성하는 자바 운영자입니다.
Instantiation: The new keyword is a Java operator that creates the object. - 초기화: 운영자 new의 뒤에 생성자에 대한 call이 오는데, 이것이 새 객체를 초기화합니다.
Initialization: The new operator is followed by a call to a constructor, which initializes the new object.
객체를 참조하는 변수 선언하기
Declaring a Variable to Refer to an Object
앞서, 변수 선언을 배운 바 있는데, 다음과 같이 씁니다:
Previously, you learned that to declare a variable, you write:
type name;
이것은 타입이 type인 데이터를 참조하기 위하여 name을 사용할 것임을 컴파일러에게 알려줍니다.
이 선언은 또한 원시변수(primitive variable)를 갖고,변수에 대한 적정한 메모리 양을 예약합니다.
또한 그 자체의 line 위에 참조 변수를 선언할 수 있습니다. 예를 들면:
This notifies the compiler that you will use name to refer to data whose type is type. With a primitive variable, this declaration also reserves the proper amount of memory for the variable.
You can also declare a reference variable on its own line. For example:
Point originOne;
위와 같이 originOne
을 선언하면, 객체가 실제로 생성되어 객체에 그 값(value)이 할당될 때까지 그 값(value)은 결정되지 않을 것입니다.
단순히 참조변수(reference variable)를 선언했다고 해서 객체가 생성되지 않습니다.
그것에 대하여, 다음 섹션에 서술된 대로 운영자 new
를 사용해야 합니다.
코드에서 사용하기 전에 객체를 참조변수 originOne
에 할당해야 합니다.
그렇지 않으면, 컴파일러 오류가 뜹니다.
If you declare originOne
like this, its value will be undetermined until an object is actually created and assigned to it. Simply declaring a reference variable does not create an object. For that, you need to use the new
operator, as described in the next section. You must assign an object to originOne
before you use it in your code. Otherwise, you will get a compiler error.
현재 객체를 참조하지 않고 있는, 이 상태에서의 참조변수는 다음 처럼 예시될 수 있습니다(참조변수이름 originOne
, 더하기 아무 것도 가리키지 않는 참조):
A variable in this state, which currently references no object, can be illustrated as follows (the variable name, originOne
, plus a reference pointing to nothing):
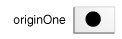
클래스 인스턴스화 하기 Instantiating a Class
운영자 new는 신규 객체에 메모리를 할당 함으로써, 해당 메모리에 참조를 리턴함으로써, 클래스를 인스턴스화 합니다.
운영자 new는 또한 객체 생성자를 invoke 합니다.
The new operator instantiates a class by allocating memory for a new object and returning a reference to that memory. The new operator also invokes the object constructor.
참고: "클래스 인스턴스화 하기"는 "객체 생성하기"와 같은 것을 의미합니다. 객체를 생성할 때, 클래스의 "인스턴스"를 생성하는 것이기 때문에 클래스를 "인스턴스화하는" 것입니다.
운영자 new는 단일의, postfix 아규먼트: 생성자에 대한 call을 요구합니다.
생성자의 이름은 인스턴화 하기 위하여 클래스의 이름을 제공합니다.
운영자 new는 그것이 생성한 객체에 대한 참조를 리턴합니다.
이 참조는 아래처럼 일반적으로 적절한 타입의 변수에 할당됩니다:
The new operator requires a single, postfix argument: a call to a constructor. The name of the constructor provides the name of the class to instantiate.
The new operator returns a reference to the object it created. This reference is usually assigned to a variable of the appropriate type, like:
Point originOne = new Point(23, 94);
운영자 new가 리턴한 참조를 반드시 변수에 할당할 필요는 없습니다.
표현식(expression) 으로 직접 사용될 수도 있기 때문입니다.
The reference returned by the new operator does not have to be assigned to a variable. It can also be used directly in an expression.
예를 들어: For example:
int height = new Rectangle().height;
This statement will be discussed in the next section.
객체 초기화하기 Initializing an Object
여기 클래스 Point에 대한 코드가 있습니다: Here's the code for the Point class:
public class Point { public int x = 0; public int y = 0; //constructor public Point(int a, int b) { x = a; y = b; } }
이 클래스에는 생성자가 1개 포함되어 있습니다.
생성자 선언은 클래스와 동일한 이름을 사용하기 때문에 그리고 생성자가 리턴 타입을 갖고 있지 않기 때문에 생성자를 인식할 수 있습니다.
클래스 Point안의 생성자는 코드 (int a, int b)가 선언한 대로 2개의 정수 아규먼트를 취합니다.
다음 statement는 그 아규먼트에 대한 값으로 23과 94를 제공합니다:
This class contains a single constructor. You can recognize a constructor because its declaration uses the same name as the class and it has no return type. The constructor in the Point class takes two integer arguments, as declared by the code (int a, int b). The following statement provides 23 and 94 as values for those arguments:
Point originOne = new Point(23, 94);
이 명령문의 실행 결과는 다음 그림 안에 예시될 수 있습니다:
The result of executing this statement can be illustrated in the next figure:
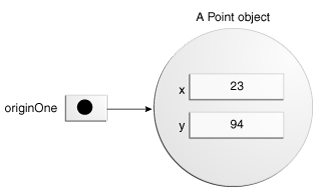
클래스 Rectangle 코드가 있는데, 이것은 4개의 생성자를 갖고 있습니다:
Here's the code for the Rectangle class, which contains four constructors:
public class Rectangle {
public int width = 0;
public int height = 0;
public Point origin;
// four constructors
public Rectangle() {
origin = new Point(0, 0);
}
public Rectangle(Point p) {
origin = p;
}
public Rectangle(int w, int h) {
origin = new Point(0, 0);
width = w;
height = h;
}
public Rectangle(Point p, int w, int h) {
origin = p;
width = w;
height = h;
}
// a method for moving the rectangle
public void move(int x, int y) {
origin.x = x;
origin.y = y;
}
// a method for computing the area of the rectangle
public int getArea() {
return width * height;
}
}
각 생성자가 원시 타입 및 참조 타입을 사용하여, rectangle의 origin, width 및 height에 대한 초기 값을 제공하게 합니다.
class가 복수의 생성자를 갖고 있다면, 그들은 서로 다른 signatures를 가져야 합니다.
자바 컴파일러는 아규먼트의 갯수 및 타입에 근거하여 생성자들을 구분합니다.
자바 컴파일러가 다음 코드를 만나면, 2개의 정수 아규먼트가 뒤따르는 아규먼트 Point를 요구하는 클래스 Rectangle 안에서 생성자를 call하는 것을 알게 됩니다:
Each constructor lets you provide initial values for the rectangle's origin, width, and height, using both primitive and reference types. If a class has multiple constructors, they must have different signatures. The Java compiler differentiates the constructors based on the number and the type of the arguments. When the Java compiler encounters the following code, it knows to call the constructor in the Rectangle class that requires a Point argument followed by two integer arguments:
Rectangle rectOne = new Rectangle(originOne, 100, 200);
이것은 origin
을 originOne
으로 초기화하는, Rectangle
의 생성자들 중 하나를 call 합니다.
또한, 생성자는 width
를 100으로 height
를 200으로 설정합니다.
이제 동일한 Point object에 대한 2개의 참조가 있습니다 - 다음 그림에서 처럼 객체는 그것에 대한 복수의 참조들을 가질 수 있습니다:
This calls one of Rectangle
's constructors that initializes origin
to originOne
. Also, the constructor sets width
to 100 and height
to 200. Now there are two references to the same Point object—an object can have multiple references to it, as shown in the next figure:
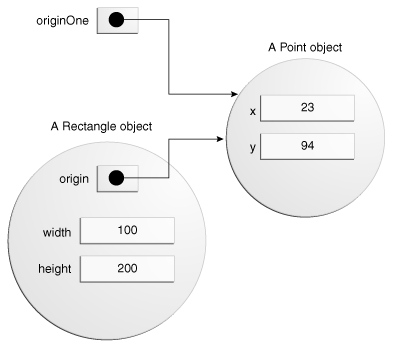
다름 라인의 코드는 2개의 아규먼트를 요구하는 생성자 Rectangle
를 call 하는데, 이것은 width 및 height에 초기 값을 제공합니다.
생성자 내의 코드를 검사한다면, 이것이 x 와 y 값이 0으로 초기화되는 new Point object를 생성하는 것을 보게 될 것입니다:
The following line of code calls the Rectangle
constructor that requires two integer arguments, which provide the initial values for width and height. If you inspect the code within the constructor, you will see that it creates a new Point object whosex and y values are initialized to 0:
Rectangle rectTwo = new Rectangle(50, 100);
다음 명령문에서 사용된 생성자 Rectangle은 아무런 아규먼트도 갖고 있지 않기 때문에, no-argument constructor라고 부릅니다
The Rectangle constructor used in the following statement doesn't take any arguments, so it's called a no-argument constructor:
Rectangle rect = new Rectangle();
모든 클래스들은 최소한 1개의 생성자를 갖고 있습니다.
클래스가 명시적으로 아무런 생성자를 선언하지 않으면, 자바 컴파일러가 default 생성자라 불리는 no-argument 생성자를 자동적으로 제공합니다.
이 default 생성자는 클래스 부모의 no-argument 생성자를 call 하거나 또는 클래스가 다른 부모를 갖고 있지 않은 경우에는 Object
생성자를 call 합니다.
부모가 생성자를 갖고 있지 않다면 (Object
는 1개를 갖고 있음), 컴파일러는 프로그램을 거절할 것입니다.
All classes have at least one constructor. If a class does not explicitly declare any, the Java compiler automatically provides a no-argument constructor, called the default constructor. This default constructor calls the class parent's no-argument constructor, or the Object
constructor if the class has no other parent. If the parent has no constructor (Object
does have one), the compiler will reject the program.
'Java 배우기' 카테고리의 다른 글
More on Classes 클래스 추가사항 (0) | 2016.01.05 |
---|---|
Using Objects 객체 사용하기 (0) | 2016.01.05 |
Objects 객체 (0) | 2016.01.05 |
Passing Information to a Method or a Constructor 메소드 또는 생성자에게 정보 전달하기 (0) | 2016.01.05 |
Providing Constructors for Your Classes 클래스에 생성자 제공하기 (0) | 2016.01.05 |
댓글