객체란 무엇인가? What Is an Object?
객체는 객체 지향 기술을 이해하는 열쇠입니다.
당장 주위를 둘러보면 현실 세계에서 많은 객체의 예를 찾을 수 있습니다:
개, 책상, 텔레비전, 자전거.
현실 세계의 객체는 2가지 특성을 공유합니다:
그들은 모두 상태와 동작을 갖고 있습니다.
개는 상태(이름, 색, 품종, 배고픔)와 동작(짖기, 지시 따르기, 꼬리 흔들기)를 갖고 있습니다.
자전거도 상태(현재의 기어, 현재의 페달 운율(cadence), 현재 속도)과 동작(기어 바꾸기, 페달 운율 바꾸기, 브레이크 밟기)을 갖고 있습니다.
현실 세계의 객체의 상태와 동작을 파악하는 것은 객체-지향 프로그래밍의 관점에서 생각을 시작하는 좋은 방법입니다.
Objects are key to understanding object-oriented technology. Look around right now and you'll find many examples of real-world objects: your dog, your desk, your television set, your bicycle.
Real-world objects share two characteristics: They all have state and behavior. Dogs have state (name, color, breed, hungry) and behavior (barking, fetching, wagging tail). Bicycles also have state (current gear, current pedal cadence, current speed) and behavior (changing gear, changing pedal cadence, applying brakes). Identifying the state and behavior for real-world objects is a great way to begin thinking in terms of object-oriented programming.
몇 분 동안 주변에 있는 현실 세계의 객체를 관찰해보십시오.
보이는 각 객체에 대하여, 스스로에게 2가지 질문을 해보십시오.
"이 객체가 어떤 상태에 있을 수 있을까요?" 그리고 "이 객체가 어떤 가능한 동작을 수행할 수 있나요?" 관찰을 기록하십시오.
관찰한 바와 같이, 현실 세계의 객체들은 복잡 다양하다는 것을 알 수 있습니다;
데스크탑 램프는 오직 2가지 상태(켜져있음과 꺼져있음)와 2가지 동작(끄기, 켜기)을 가질 수 있지만, 데스크탑 라디오는 추가적인 상태(켜져있음, 꺼져있음, 현재 볼륨, 현재 방송국)와 동작(켜기, 끄기, 볼륨 높이기, 볼륨 낮추기, 찾기, 스캔과 조정)를 가질 수 있습니다.
또한 일부 객체는, 차례로, 다른 객체들을 포함할 수 있다는 것도 알 수 있습니다.
이러한 현실 세계에 대한 관찰은 모든 것을 객체-지향 프로그래밍의 세계로 변환시킵니다.
Take a minute right now to observe the real-world objects that are in your immediate area. For each object that you see, ask yourself two questions: "What possible states can this object be in?" and "What possible behavior can this object perform?". Make sure to write down your observations. As you do, you'll notice that real-world objects vary in complexity; your desktop lamp may have only two possible states (on and off) and two possible behaviors (turn on, turn off), but your desktop radio might have additional states (on, off, current volume, current station) and behavior (turn on, turn off, increase volume, decrease volume, seek, scan, and tune). You may also notice that some objects, in turn, will also contain other objects. These real-world observations all translate into the world of object-oriented programming.
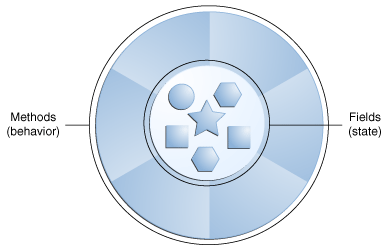
A software object.
소프트웨어 객체들은 개념적으로 현실 세계의 객체들과 유사합니다:
소프트웨어 객체들 역시 상태와 관련 동작으로 구성되어 있습니다.
하나의 객체는 필드(fields, 일부 프로그래밍 언어에서는 변수(variables))에 그 상태를 저장하고, 메소드(methods, 일부 프로그래밍 언어에서는 함수(functions))를 통해 그 동작을 노출합니다.
메소드들은 객체의 내부 상태(internal state) 위에서 작동하며, 객체간 커뮤니케이션을 위한 기본 메커니즘 역할을 합니다.
내부 상태를 숨기고 모든 상호작용을 객체의 메소드를 통하여 수행하는 것이 데이터 캡슐화(객체-지향 프로그래밍의 기본 원칙)입니다 .
Software objects are conceptually similar to real-world objects: they too consist of state and related behavior. An object stores its state in fields (variables in some programming languages) and exposes its behavior through methods (functions in some programming languages). Methods operate on an object's internal state and serve as the primary mechanism for object-to-object communication. Hiding internal state and requiring all interaction to be performed through an object's methods is known as data encapsulation — a fundamental principle of object-oriented programming.
예를 들어 자전거를 생각해봅니다:
Consider a bicycle, for example:
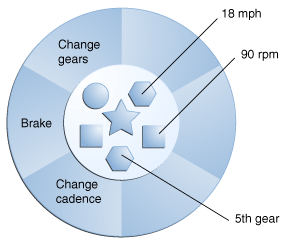
소프트웨어 객체로 모델된 자전거 A bicycle modeled as a software object.
상태(현재 속도, 현재 페달 운율, 현재 기어)를 부여하고 그 상태를 변경하는 메소드를 제공함으로써, 외부세계는 메소드를 사용하여 객체를 제어할 수 있습니다.
예를 들어 자전거가 기어를 6개만 가진 경우, 기어를 변경하는 메소드는 1보다 작거나 6보다 큰 값을 거절할 수 있습니다.
코드를 개별 소프트웨어 객체들로 묶으면 아래와 같은 많은 효과를 볼 수 있습니다:
By attributing state (current speed, current pedal cadence, and current gear) and providing methods for changing that state, the object remains in control of how the outside world is allowed to use it. For example, if the bicycle only has 6 gears, a method to change gears could reject any value that is less than 1 or greater than 6.
Bundling code into individual software objects provides a number of benefits, including:
1. 모듈화(Modularity): 객체 별 소스 코드를 작성할 수 있고, 그 코드를 다른 객체들과 독립적으로 유지할 수 있습니다. 객체가 일단 만들어지면, 그 객체는 시스템 내부 주위에 쉽게 전달될 수 있습니다.
The source code for an object can be written and maintained independently of the source code for other objects. Once created, an object can be easily passed around inside the system.
2. 정보 은폐(Information-hiding): 객체의 메소드들과만 상호작용하여, 객체 내부의 세부 구현사항은 외부세계에 대하여 숨겨진 상태로 유지됩니다.
By interacting only with an object's methods, the details of its internal implementation remain hidden from the outside world.
3. 코드 재사용(Code re-use): (아마도 다른 소프트웨어 개발자가 작성한) 객체가 이미 존재하는 경우, 프로그램에서 그 객체를 사용할 수 있습니다.
이에 따라 전문가들이 복잡하고, 과제-특정 객체들을 구현/테스트/디버그 할 수 있는데, 그러면 각자가 자신의 코드에서 이러한 객체를 실행하는 것을 신뢰할 수 있습니다.
If an object already exists (perhaps written by another software developer), you can use that object in your program. This allows specialists to implement/test/debug complex, task-specific objects, which you can then trust to run in your own code.
4. 플러그 인 및 디버깅을 쉽게(Pluggability and debugging ease): 특정 객체가 문제있는 것으로 판명되면, 단순히 애플리케이션에서 그 객체를 제거하고 그 대체로 다른 객체를 플러그(plug) 할 수 있습니다.
이것은 현실 세계에서 기계적인 문제를 해결하는 것과 유사합니다.
볼트가 깨진 경우, 전체 기계 대신 볼트만 교체하지요.
If a particular object turns out to be problematic, you can simply remove it from your application and plug in a different object as its replacement. This is analogous to fixing mechanical problems in the real world. If a bolt breaks, you replace it, not the entire machine.
'Java 배우기' 카테고리의 다른 글
What Is Inheritance? 상속이란 무엇인가? (0) | 2016.01.05 |
---|---|
What Is a Class? 클래스란 무엇인가? (0) | 2016.01.05 |
Lesson: Object-Oriented Programming Concepts 단원: 객체-지향 프로그래밍 (0) | 2016.01.05 |
Trail: Learning the Java Language 자바 언어 배우기 및 자바 다운로드 받기 (0) | 2016.01.05 |
What Can Java Technology Do? 자바 기술로 할 수 있는 일 (0) | 2016.01.05 |
댓글