메소드로부터 값을 리턴하기 Returning a Value from a Method
메소드가 다음을 하는 경우, 어떤 것이든 먼저 발생되는 경우, 메소드는 그것을 invoke한 코드를 리턴합니다
A method returns to the code that invoked it when it
- 메소드 안의 모든 명령문(statements)을 완료한 경우
return
명령문에 도달한 경우, 또는- reaches a
return
statement, 또는 인 경우 리턴 스테이드 먼드ㅔ - exception을 throw 한 경우(후에 다룸)
throws an exception (covered later),
메소드 선언 안에 메소드의 return type을 선언합니다.
메소드의 몸체 내에서, 값을 리턴하기 위하여 return
명령문을 사용합니다.
void
로 선언된 모든 메소드는 값을 리턴하지 않습니다.
void 로 선언된 모든 메소드는 return
명령문을 포함할 필요가 없지만, 포함할 수도 있습니다.
그러한 경우, return
명령문은 control flow block을 branch out 한 다음, 메소드를 exit하는데 사용될 수 있으며 아래와 같이 간단하게 사용됩니다:
whichever occurs first.
You declare a method's return type in its method declaration. Within the body of the method, you use the return
statement to return the value.
Any method declared void
doesn't return a value. It does not need to contain a return
statement, but it may do so. In such a case, a return
statement can be used to branch out of a control flow block and exit the method and is simply used like this:
return;
void로 선언된 메소드로부터 값을 리턴하려 하면 컴파일러 오류가 생깁니다.
void로 선언되지 않은 모든 메소드는 상응하는 리턴 값을 가진 return statement를 아래와 같이 포함해야 합니다.
If you try to return a value from a method that is declared void
, you will get a compiler error.
Any method that is not declared void
must contain a return
statement with a corresponding return value, like this:
return returnValue;
리턴 값의 데이터타입은 메소드가 선언한 리턴 타입과 일치해야 하며; boolean을 리턴하도록 선언된 메소드 로부터 정수 값을 리턴할 수는 없습니다.객체관련하여 이 섹션에서 논의되었던 Rectangle
Rectangle
class내의 getArea()
method는 integer를 리턴합니다:
The data type of the return value must match the method's declared return type; you can't return an integer value from a method declared to return a boolean.
The getArea()
method in the Rectangle
Rectangle
class that was discussed in the sections on objects returns an integer:
// rectangle의 면적 계산 메소드 public int getArea() { return width * height; }
이 method는 expression width*height
가 evaluate하는 정수를 리턴합니다.
getArea
method는 primitive type을 리턴합니다.
method는 reference type도 리턴할 수 있습니다.
예를 들어, 프로그램에서 Bicycle
objects을 조작하기 위하여, 아래와 같은 메소드를 가질 수도 있습니다:
This method returns the integer that the expression width*height
evaluates to.
The getArea
method returns a primitive type. A method can also return a reference type. For example, in a program to manipulate Bicycle
objects, we might have a method like this:
public Bicycle seeWhosFastest(Bicycle myBike, Bicycle yourBike,
Environment env) {
Bicycle fastest;
// 각각의 자전거의 기어와 cadence, 그리고
// 환경(terrain 및 바람)이 주어진 상황에서
// 어떤 자전거가 빠른지 계산하기 위한 코드
return fastest;
}
클래스 또는 인터페이스 리턴하기 Returning a Class or Interface
이 섹션이 혼동되면 이것을 건너뛰고 인터페이스 및 상속에 관한 단원을 마친 다음 다시 돌아와도 됩니다.
If this section confuses you, skip it and return to it after you have finished the lesson on interfaces and inheritance.
whosFastest
가 하는 것처럼 메소드가 그 리턴 타입으로 클래스 이름을 사용하는 경우, 리턴된 객체의 타입의 클래스는 리턴 타입의 서브클래스이거나, 또는 리턴 타입과 정확하게 같은 클래스이어야 합니다.
다음 그림에 예시된 대로 ImaginaryNumber
가 java.lang.Number
의 서브클래스인 클래스 계층구조를 갖고 있다고 상정하면, 이것은 순서에 따라 Object
의 서브클래스 입니다.
When a method uses a class name as its return type, such as whosFastest
does, the class of the type of the returned object must be either a subclass of, or the exact class of, the return type. Suppose that you have a class hierarchy in which ImaginaryNumber
is a subclass of java.lang.Number
, which is in turn a subclass of Object
, as illustrated in the following figure.
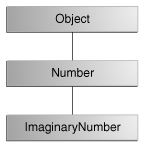
The class hierarchy for ImaginaryNumber
Number을 리턴하도록 선언된 메소드가 있다고 가정합니다:
Now suppose that you have a method declared to return a Number
:
public Number returnANumber() {
...
}
returnANumber
method는 ImaginaryNumber
를 리턴할 수 있지만 Object
는 리턴할 수 없습니다.ImaginaryNumber
는 Number
의 서브클래스 이기 때문에 Number
입니다.
하지만, Object
는 반드시 Number
는 아니고— String
또는 다른 타입이 될 수도 있습니다.
메소드를 override 해서 원래 메소드의 서브클래스를 리턴하도록 아래와 같이 정의할 수 있습니다:
The returnANumber
method can return an ImaginaryNumber
but not an Object
. ImaginaryNumber
is a Number
because it's a subclass of Number
. However, an Object
is not necessarily a Number
— it could be a String
or another type.
You can override a method and define it to return a subclass of the original method, like this:
public ImaginaryNumber returnANumber() {
...
}
covariant return type이라 불리는 이 기법은 return type이 subclass와 같은 방향으로 변형될 수 있음을 의미합니다.
This technique, called covariant return type, means that the return type is allowed to vary in the same direction as the subclass.
Note: You also can use interface names as return types. In this case, the object returned must implement the specified interface.
'Java 배우기' 카테고리의 다른 글
Controlling Access to Members of a Class 클래스 멤버들에 대한 접근 제어 (0) | 2016.01.05 |
---|---|
Using the this Keyword 키워드 this 사용하기 (0) | 2016.01.05 |
More on Classes 클래스 추가사항 (0) | 2016.01.05 |
Using Objects 객체 사용하기 (0) | 2016.01.05 |
Creating Objects 객체 생성하기 (0) | 2016.01.05 |
댓글